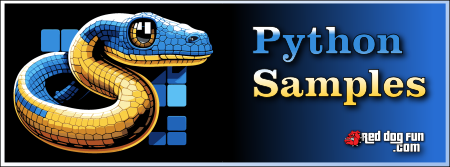
Here’s a list of the standard Python modules that are included with Python:
abc
: Abstract Base Classesargparse
: Command-line Argument Parsingarray
: Efficient arrays of numeric valuesasyncio
: Asynchronous I/O, event loop, coroutines and tasksatexit
: Exit handlersbase64
: Base16, Base32, Base64, Base85 Data Encodingsbisect
: Array bisection algorithmbuiltins
: Built-in objectscProfile
: Deterministic profiling of Python programscalendar
: General calendar-related functionscgi
: Common Gateway Interface supportcgitb
: Traceback manager for CGI scriptschunk
: Tools for iterating over iterables by chunkcmath
: Mathematical functions for complex numberscmd
: Support for line-oriented command interpreterscodecs
: Codec registry and base classescodeop
: Compile (possibly incomplete) Python codecollections
: Container datatypescolorsys
: Conversions between color systemscompileall
: Byte-compile Python source filesconcurrent
: Executors for concurrent.futuresconfigparser
: Configuration file parsercontextlib
: Utilities for with-statement contextscontextvars
: Context Variablescopy
: Shallow and deep copy operationscopyreg
: Register pickle support functionscrypt
: Functionality for one-way hashing of datacsv
: CSV File Reading and Writingctypes
: A foreign function library for Pythondataclasses
: Data Classesdatetime
: Basic date and time typesdecimal
: Decimal fixed point and floating point arithmeticdifflib
: Helpers for computing deltasdis
: Disassembler for Python bytecodedistutils
: Building and installing Python modulesdoctest
: Test interactive Python examplesemail
: Package supporting the parsing, manipulation, and generating of email messagesencodings
: Encoding and decoding of stringsenum
: Support for enumerationserrno
: Standard errno system symbolsfaulthandler
: Dump the Python tracebackfcntl
: The fcntl and ioctl system callsfilecmp
: File and Directory Comparisonsfileinput
: Iterate over lines from multiple input streamsfnmatch
: Unix-style filename pattern matchingformatter
: Generic output formattingfractions
: Rational Numbersftplib
: FTP protocol clientfunctools
: Higher-order functions and operations on callable objectsgc
: Garbage Collector interfacegetopt
: C-style parser for command line optionsgetpass
: Portable password inputgettext
: Multilingual internationalization servicesglob
: Unix style pathname pattern expansiongrp
: Group Database (getgrnam() and friends)gzip
: Support for gzip fileshashlib
: Secure hashes and message digestsheapq
: Heap queue algorithmhmac
: Keyed-Hashing for Message Authenticationhtml
: HyperText Markup Language supporthttp
: HTTP protocol client and serverimaplib
: IMAP4 protocol clientimghdr
: Determine the type of an image fileimp
: Deprecated, Importing modulesimportlib
: A powerful and flexible importer mechanisminspect
: Inspect live objectsio
: Core tools for working with streamsipaddress
: IPv4/IPv6 manipulation libraryitertools
: Functions creating iterators for efficient loopingjson
: JSON encoder and decoderkeyword
: Testing for Python keywordslib2to3
: Automated Python 2 to 3 code translationlinecache
: Random access to text lineslocale
: Internationalization serviceslogging
: Flexible event logging system for applicationslzma
: Compression using the LZMA algorithmmailbox
: Manipulate mailboxes in various formatsmailcap
: Mailcap file handlingmarshal
: Internal Python object serializationmath
: Mathematical functions (sin() etc.)mimetypes
: Map filenames to MIME typesmmap
: Memory-mapped file supportmodulefinder
: Find modules used by a scriptmultiprocessing
: Process-based parallelismnetrc
: Read netrc filesnntplib
: NNTP protocol clientnumbers
: Numeric abstract base classesopcode
: Operations codes used by the Python interpreteroptparse
: Deprecated since version 3.2: Use argparse insteados
: Miscellaneous operating system interfacesossaudiodev
: Access to OSS-compatible audio devicesparser
: Access parse trees for Python source codepathlib
: Object-oriented filesystem pathspdb
: The Python Debuggerpickle
: Python object serializationpickletools
: Tools for working with Python picklespipes
: Interface to shell pipelinespkgutil
: Package extension utilityplatform
: Platform identificationplistlib
: Generate and parse Mac OS X .plist filespoplib
: POP3 protocol clientpprint
: Data pretty printerprofile
: Deterministic profiling of Python programspstats
: Statistics object for use with the profilerpty
: Pseudo-Terminal Handling for Linuxpwd
: Password database (getpwnam() and friends)py_compile
: Compile Python source files to bytecodequeue
: A synchronized queue classquopri
: Encode and decode MIME quoted-printable datarandom
: Generate pseudo-random numbersre
: Regular expression operationsreadline
: GNU readline supportreprlib
: Alternate repr() implementationresource
: Resource usage informationrlcompleter
: Completion function for GNU readlinerunpy
: Locating and running Python modulessched
: Event schedulersecrets
: Generate secure random numbers for managing secretsselect
: Waiting for I/O completionshelve
: Python object persistenceshlex
: Simple lexical analysisshutil
: High-level file operationssignal
: Set handlers for asynchronous eventssite
: Site-specific configuration hooksmtpd
: SMTP server classessmtplib
: SMTP protocol clientsndhdr
: Determine type of sound filesocket
: Low-level networking interfacesocketserver
: A framework for network serverssqlite3
: DB-API 2.0 interface for SQLite databasesssl
: TLS/SSL wrapper for socket objectsstat
: Interpreting stat() resultsstatistics
: Mathematical statistics functionsstring
: Common string operationsstringprep
: Internet string preparationstruct
: Interpret bytes as packed binary datasubprocess
: Subprocess managementsunau
: Read and write Sun AU filessymbol
: Constants used with Python parse treessymtable
: Access to the symbol tables used by the Python compilersys
: System-specific parameters and functionssysconfig
: Access Python’s configuration informationtabnanny
: Indentation validatortarfile
: Read and write tar archive filestelnetlib
: Telnet clienttempfile
: Generate temporary files and directoriestermios
: POSIX terminal controltest
: Support for unit testingtextwrap
: Text wrapping and fillingthis
: Prints the Zen of Pythonthreading
: Thread-based parallelismtime
: Time access and conversionstimeit
: Measure execution time of small code snippetstkinter
: Python interface to Tcl/Tktoken
: Constants representing terminal nodes of Python parse treestokenize
: Tokenize Python source codetrace
: Trace or track Python statement executiontraceback
: Print or retrieve a stack tracebacktracemalloc
: Debug tools for tracking memory allocationstty
: Terminal control functionsturtle
: Turtle graphicsturtledemo
: Demonstration suite for the turtle graphics moduletypes
: Dynamic type creation and names for built-in typestyping
: Support for type hintsunicodedata
: Unicode Databaseunittest
: Unit testing frameworkurllib
: URL handling modulesuu
: UUencode and UUdecode filesuuid
: UUID objects according to RFC 4122venv
: Creation of virtual environmentswarnings
: Warning controlwave
: Read and write WAV filesweakref
: Weak referenceswebbrowser
: Convenient Web-browser controllerwsgiref
: WSGI Utilities and Reference Implementationxdrlib
: Encode and decode XDR dataxml
: Package containing XML processing modulesxmlrpc
: XML-RPC server and client moduleszipapp
: Manage executable Python zip archiveszipfile
: Work with ZIP archiveszipimport
: Import modules from ZIP archives.zlib
: Compression and decompression using the zlib library.
It’s worth noting that not all of these modules are available on all platforms, and some of them may have platform-specific behaviors. Additionally, Python has an extensive library of third-party modules available for download, which can greatly expand its capabilities.
Please follow and like us:
Leave a reply
You must be logged in to post a comment.