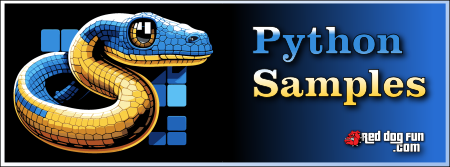
Introduction
In programming, a variable is a container that holds a value. The value can be a number, text, or any other type of data. Variables are useful because they allow you to store and manipulate data in your programs.
Naming Variables
To create a variable in Python, you first need to give it a name. The name of a variable should be descriptive and meaningful. It should also follow a few rules:
- The name can only contain letters, numbers, and underscores.
- The name cannot start with a number.
- The name should not be a Python keyword, such as
if
,else
, orfor
.
Here are some examples of valid variable names:
python |
age = 42 name = “John” is_student = True |
Assigning Values to Variables
After you have named your variable, you can assign a value to it using the equal sign (=
). Here are some examples:
python |
x = 10 y = 3.14 z = “Hello, World!” |
In the first example, x
is assigned the value 10
. In the second example, y
is assigned the value 3.14
. In the third example, z
is assigned the value "Hello, World!"
.
Data Types
Python has several data types, including:
- Integer (
int
): A whole number, such as42
. - Float (
float
): A decimal number, such as3.14
. - String (
str
): A sequence of characters, such as"Hello, World!"
. - Boolean (
bool
): A value that is eitherTrue
orFalse
.
When you assign a value to a variable, Python automatically determines its data type based on the value. For example, in the following code, x
is an int
and y
is a float
:
python |
x = 10 y = 3.14 |
Printing Variables
To see the value of a variable, you can use the print()
function. Here’s an example:
python |
age = 42 print(age) |
This will output 42
to the console.
Changing Variable Values
You can change the value of a variable by assigning a new value to it. Here’s an example:
python |
age = 42 print(age) # Output: 42 age = 43 print(age) # Output: 43 |
In the first print()
statement, age
is 42
. After assigning a new value of 43
, the second print()
statement outputs 43
.
Conclusion
Variables are an essential part of programming in Python. They allow you to store and manipulate data in your programs. By following the rules for naming variables and assigning values to them, you can use variables to create powerful and flexible code.
Leave a reply
You must be logged in to post a comment.